Плата центрального недопроцессора nedoCPU-32
Moderator: Shaos
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Вот совмещения цветов с белым-чёрным-серым на большом современном телеке:
Также я добавил 2 узкие серые полоски - причём левый серый это 0101 (5), а правый - 1010 (10). Тут видно, что некоторые цвета достаточно гладко стыкуются с чёрным, а некоторые с белым. Серый лучше всего стыкуется с белым и чёрным, давая чёткость 320x200 (а чёткость чёрно-белых границ по сути 640х200) - выходит можно делать вставки высокой чёткости с градациями серого из 3 цветов (и ещё более высокой чёткости чёрные объекты на белом фоне либо белые объекты на чёрном).
Также я добавил 2 узкие серые полоски - причём левый серый это 0101 (5), а правый - 1010 (10). Тут видно, что некоторые цвета достаточно гладко стыкуются с чёрным, а некоторые с белым. Серый лучше всего стыкуется с белым и чёрным, давая чёткость 320x200 (а чёткость чёрно-белых границ по сути 640х200) - выходит можно делать вставки высокой чёткости с градациями серого из 3 цветов (и ещё более высокой чёткости чёрные объекты на белом фоне либо белые объекты на чёрном).
You do not have the required permissions to view the files attached to this post.
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
не выходит, если есть отдельно стоящие серые полупикселы - телек "увидит" в них цвет:Shaos wrote:...выходит можно делать вставки высокой чёткости с градациями серого из 3 цветов...
а вот если совсем отключить цвет, то будет виден каждый чёрно-белый пиксел в полном разрешении 640х200:
You do not have the required permissions to view the files attached to this post.
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Попробовал как естьShaos wrote:Надо чтоли вспомнить как PIC32 программится да прошивается и уже попробоватьShaos wrote:Может мне сделать официальный видеорежим с таким фейковым интерлейсом? Хотя бы чёрно-белый 640x400 (ядро само по прерыванию будет переключать видеобуфера) - и то куда веселее будет (разбавленным дизерингом чёрно-белым полутоновым изображениям по сути не важно в каком порядке идут чётные и нечётные строки)? Вот например что можно будет показать в таком режиме на экране ТВ:
P.S. Оригинал картинки бобинника можно поглядеть вот тут
P.P.S. Для защиты от перепутывания чётных и нечётных строк можно делать не монохром 640x400, а 5 градаций серого в 320x200 - тогда можно каждый пиксел заменить на квадрат 2х2 (с потерей чёткости):(также дописал справа вариант в случае перестановки чётных и нечётных строк местами - так понятнее будет что я имею ввиду)Code: Select all
0x0 -> 0 0 = 0 0 0 0 0 0 0x1 -> 0 0 = 0 1 0 1 0 0 0x2 -> 1 0 = 0 1 0 1 1 0 0x3 -> 1 1 = 0 1 0 1 1 1 0x4 -> 1 1 = 1 1 1 1 1 1
P.P.P.S. Либо сохранить высокое горизонтальное разрешение ограничившись ТРЕМЯ градациями серого для пиксела в картинке разрешением 640x200 и делать "интеллектуальный" дизеринг, который будет учитывать накапливаемую по ходу строки ошибку и учитывать её при подгоне следующих двоек монохромных пикселов...

Вот более новая тестовая картинка 640x400:
Напомню, что высокое разрешение тут получено "мельтешением" двух полукадров с частотой смены строк (большинство современных телеков раскладывают соседние полукадры в полный кадр даже если это не интерлейсный сигнал) - однако с вероятностью 50% телек может начать с неправильного полукадра тогда чётные и нечётные строки переставятся:
Поэтому таким способом надо представлять только такие картинки в которых неважен порядок чётных-нечётных строк...
P.S. А вот что будет если включить цвет:
You do not have the required permissions to view the files attached to this post.
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Надо вспомнить как я планировал добавлять кнопки и звук...Shaos wrote:Переставил синхру с PortB.5 на PortB.1, освободив тем самым 8 контактов подряд для кнопок - от PortB.4 до PortB.11
...
А на PortB.2 и PortB.3 можно выдавать 2-битный звук с частотой дискретизации 15734.2 Гц (частота строчной развёртки NTSC)...
...
С другой стороны зачем 2-битный звук, если можно сделать ШИМ на блоке Compare, которых ещё осталось?...
P.S. Подцепил 2 Compare - один будет ШИМ на звук, а второй - на подсветку отдельных строк
P.S. В digikey.com всё ещё есть 300 штук 50-мегагерцовых PIC32 в DIP28 за $4.98 (если брать 100 штук):
https://www.digikey.com/en/products/detail/microchip-technology/PIC32MX170F256B-50I-SP/4902612
You do not have the required permissions to view the files attached to this post.
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
А пока вот ещё одна картинка 640x400 на экране большого телека Hisense с RokuOS (картинка подготовлена для соотношения сторон 16:9):
You do not have the required permissions to view the files attached to this post.
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Xorya показывает собственную принципиальную схему на экране маленького телевизора! 

You do not have the required permissions to view the files attached to this post.
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Supreme God
- Posts: 16720
- Joined: 21 Oct 2009 08:08
- Location: Россия
Re: Плата центрального недопроцессора nedoCPU-32
А ты полу-строку делаешь? Насколько я помню, поля (полукадры) отличаются полу-строкой.Shaos wrote:...большинство современных телеков раскладывают соседние полукадры в полный кадр даже если это не интерлейсный сигнал) - однако с вероятностью 50% телек может начать с неправильного полукадра тогда чётные и нечётные строки переставятся:
В одном из полей первая строка начинается по центру, и также по центру последняя строка заканчивается.
iLavr
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Нет не делаю - телеки сами склеивают (полустрока видимо только для маркировки первого полукадра из двух нужна, чтобы порядок не путался).
Впервые я такое поведение современных телеков заметил на плоской соне (которая у меня был с ноября 2006 по июль 2020) примерно в 2016 году, когда игрался с Timex Sinclair.
Все новые телеки Roku, что у меня есть по работе, тоже так делают (более того, они ещё и развёртку PAL умеют держать т.е. у них чипсет универсальный).
Маленький телек CRAIG (выше на фотке) не склеивает, а мерцает, выводя каждый кадр без склейки с предыдущим - видимо также будут делать и старые телеки с ЭЛТ (таких тут практически нет уже).
Есть ещё небольшой телек INSIGNIA, который тоже склеивает, однако у него другая напасть - если отключить цвет (color burst гребёнки в начале каждой строки), то он не распознаёт NTSC сигнал вообще и показывает чёрный экран...
Впервые я такое поведение современных телеков заметил на плоской соне (которая у меня был с ноября 2006 по июль 2020) примерно в 2016 году, когда игрался с Timex Sinclair.
Все новые телеки Roku, что у меня есть по работе, тоже так делают (более того, они ещё и развёртку PAL умеют держать т.е. у них чипсет универсальный).
Маленький телек CRAIG (выше на фотке) не склеивает, а мерцает, выводя каждый кадр без склейки с предыдущим - видимо также будут делать и старые телеки с ЭЛТ (таких тут практически нет уже).
Есть ещё небольшой телек INSIGNIA, который тоже склеивает, однако у него другая напасть - если отключить цвет (color burst гребёнки в начале каждой строки), то он не распознаёт NTSC сигнал вообще и показывает чёрный экран...
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Всё что касается точной эмуляции композитного сигнала NTSC на PC перенёс в топик про XORLib:
viewtopic.php?f=81&t=10948
viewtopic.php?f=81&t=10948
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Я за эти 8 лет посеял где-то все эти тестовые картинки - видимо придётся заново генерить, в частности вот эту:
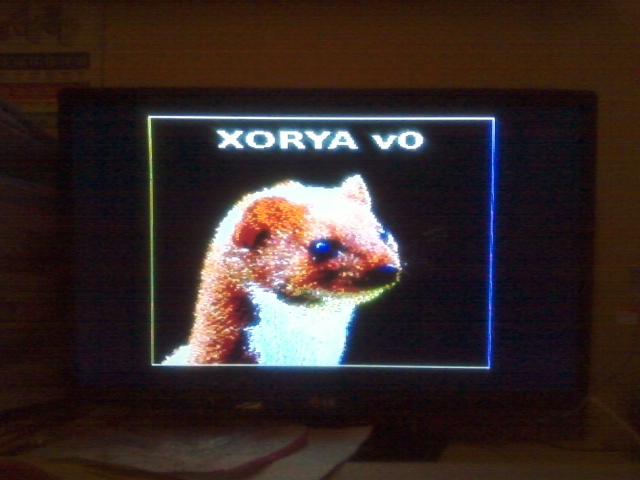
Чтобы её сделать я использовал картинку из википедии - она под CC BY-SA 2.0
https://en.wikipedia.org/wiki/Weasel#/media/File:Mustela_nivalis_-British_Wildlife_Centre-4.jpg

Испаноязычные часто читают X как "хэ" ну и Хоря = хорёк
Чтобы её сделать я использовал картинку из википедии - она под CC BY-SA 2.0
https://en.wikipedia.org/wiki/Weasel#/media/File:Mustela_nivalis_-British_Wildlife_Centre-4.jpg
P.S. Кстати может быть тогда стандартным произношением сделать "хоря", а не "зоря"Least Weasel (Mustela nivalis) at the British Wildlife Centre, Surrey, England - photograph taken Sunday 17. August 2008.
Keven Law https://flickr.com/people/66164549@N00
https://flickr.com/photos/66164549@N00/2771844162

Испаноязычные часто читают X как "хэ" ну и Хоря = хорёк

Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Вроде всё нашёл на хард-драйве снятом с давно помершего WinXP ноута, с которым я учился в New York University с 2012 по 2018 годы и на котором делал кое-какие свои проекты в те годы...Shaos wrote:Я за эти 8 лет посеял где-то все эти тестовые картинки - видимо придётся заново генерить, в частности вот эту
You do not have the required permissions to view the files attached to this post.
Я тут за главного - если что шлите мыло на me собака shaos точка net
-
- Junior
- Posts: 1
- Joined: 06 Sep 2024 02:36
Re: Плата центрального недопроцессора nedoCPU-32
Я смотрел на старые Ваши записи, ссылки были на github.com/shaos/...
а там сейчас только свежие с 2021 что-ли года.
а там сейчас только свежие с 2021 что-ли года.
-
- Admin
- Posts: 24129
- Joined: 08 Jan 2003 23:22
- Location: Silicon Valley
Re: Плата центрального недопроцессора nedoCPU-32
Я в июне 2018 перенёс все свои софтовые проекты с гитхаба на https://gitlab.com/shaos/
На гитхабе только хардверные лежат (чипы и т.д.) - кстати я там же написал в описании, что переехал (даже 2 раза):
На гитхабе только хардверные лежат (чипы и т.д.) - кстати я там же написал в описании, что переехал (даже 2 раза):
You do not have the required permissions to view the files attached to this post.
Я тут за главного - если что шлите мыло на me собака shaos точка net